Things that won’t work using Vue
After using Vue for a while, I have found some issues that later I learned are actually things they warned us in the documentation.
I made a list of these issues so you can know them as well. Let’s describe each and see the options we have to solve them.
Array Changes
Let’s say we have this:
const vm = new Vue({
data: {
titles: ['Ready Player One', 'The Power of Less', 'The 10x rule']
}
})
We can get two different issues while trying to do changes to titles
:
- Setting an item using the index:
vm.titles[index] = newTitle
- Modifying the length:
vm.titles.length = length
Instead of setting an item using the index directly you can use Vue.set
:
Vue.set(vm.titles, index, newTitle)
Another alternative is using splice
:
vm.titles.splice(index, 1, newTitle)
On the other hand, to modify the length you can use splice
as well:
vm.titles.splice(length)
Adding properties to an object
Having the fowlloing object:
const vm = new Vue({
data: {
top: {
bestMovie: 'Avengers: Infinity War'
}
}
})
We could be tempted to add a new property doing the next:
vm.top.bestShow = 'Breaking Bad'
but then bestShow
won’t be reactive because Vue adds the reactive functionality at the moment of the initialization. Which means that the property must be in the data
object in order to be reactive.
We can use Vue.set
again to accomplish this:
Vue.set(vm.top, 'bestShow', 'Breaking Bad')
Although, if you need to add several properties, maybe would be better to create a fresh object combining them:
vm.top = Object.assign({}, vm.top, { bestShow: 'Breaking Bad', bestBook: 'Ready Player One' })
Using $refs
before the component is mounted
Let’s say we want to focus an input on our component. We could use the ref
attribute for that:
<input ref="input" />
and then:
methods: {
focusInput: function () {
this.$refs.input.focus()
}
}
What if we want to focus it as soon as the component is created? You would say that we could use the created
hook:
created() {
this.$refs.input.focus()
}
but actually this produces an error because $refs
is populated after the component has been mounted. So, we should use the mounted
hook instead since it will be already populated:
mounted() {
this.$refs.input.focus()
}
Toggling similar elements
Vue sometimes will reuse elements that have the same tag name when using v-if
on them. As you can see in the next example, the input element is reused when the toggle button is pressed.
To notice this you can write something in the input and then press the button (the value won’t change) or you can use the dev tools and see that the element is not replaced:
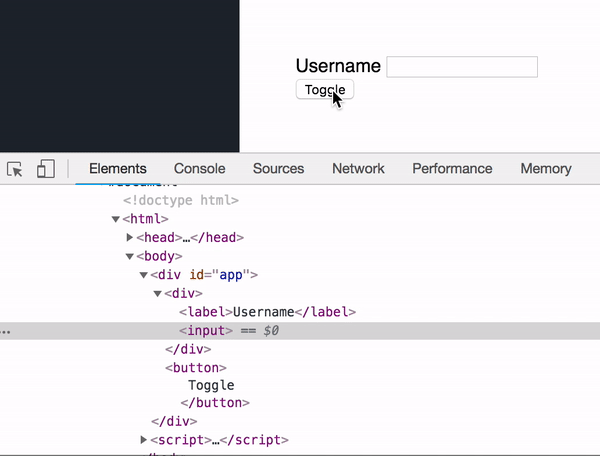
To solve this we just need to add a key to each of them so Vue knows they are distinct elements:
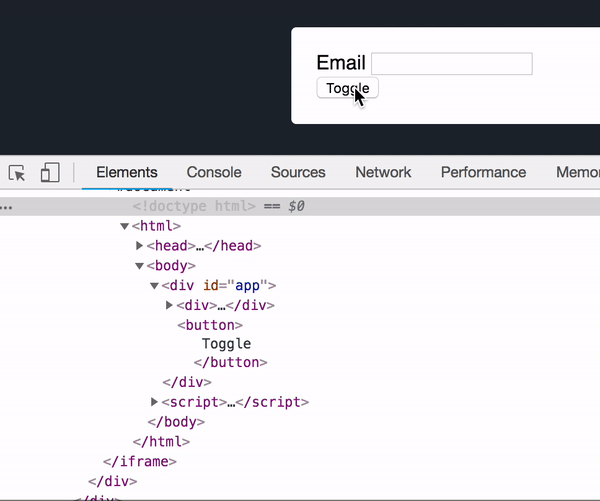
Now we can see that the element is replaced every time we click the button.
Have you noticed other things that won’t work on Vue? Please share in the comments.
Leave a Comment