What is CORS?
Have you ever seen this error?
If you have, it probably means that you have faced this problem before or that you are facing it right now. Either way, don’t worry, we will learn what is this error about together.
First of all, CORS stands for Cross-Origin Resource Sharing and I think that with this alone we could already start to understand the idea behind CORS. It is a mechanism to allows (or not) different origins (different websites, domains) to have access to resources on a server.
Let’s say that I have a service called musicinfo.com
. Here I have 2 endpoints: one that is public, meaning anyone can do requests from any origin and get information about a song, and another one that is private, meaning that only requests made from https://www.musicinfo.com
.
This is how those request would look like:
Request Headers
... Other headers
authority: www.musicinfo.com
method: GET
path: /song?name=one&artist=u2
origin: https://winnercrespo.com
Response Headers
... Other headers
Access-Control-Allow-Origin: *
As you can see, on the response we get Access-Control-Allow-Origin: *
meaning that any origin is allowed to access this resource. Another way this could have worked is if we get Access-Control-Allow-Origin: https://winnercrespo.com
meaning that this origin has access. What we get on the Access-Control-Allow-Origin
is dictated by the server so it decides beforehand who has access to what resource.
On the other hand, if I try to do a request to the private endpoint from https://winnercrespo.com we would get a CORS error as we can see in the next example.
Using the Google Seach, I noticed that it does a Get
request to this URL https://www.google.com/complete/search?q=hola
in order to get the autocomplete options.
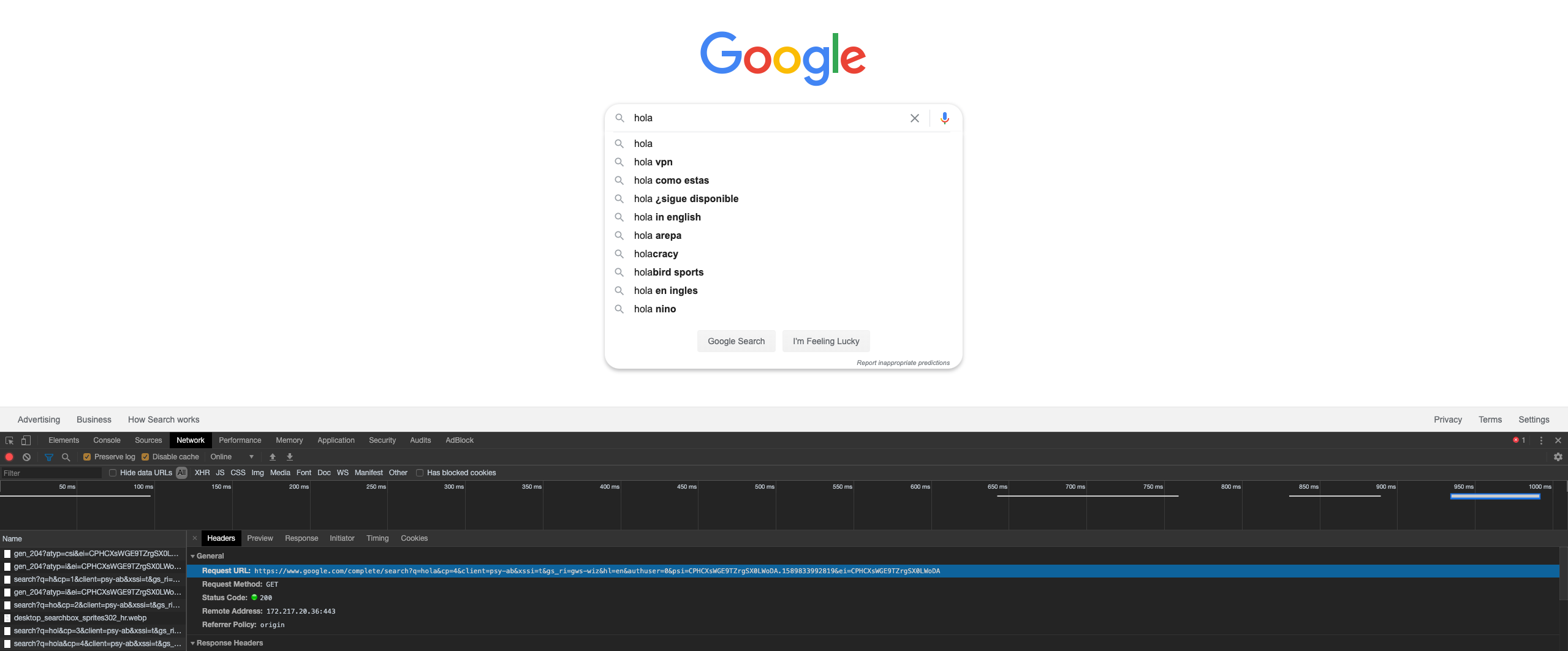
But if I try to do the same request from different website with a piece of code similar to this:
fetch('https://www.google.com/complete/search?q=hola')
.then(() => {
// handle success
})
.catch(() => {
// handle exception
});
I get the same error we saw before:
To learn more about this topic I would recommend this great post https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS written by the MDN web docs.
Leave a Comment